Java HTTP
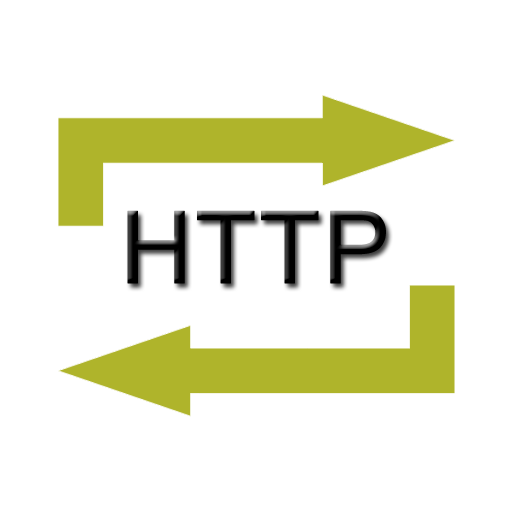
A Java utility library that makes HTTP requests easier to work with.
The Java-HTTP library simplifies the creation and execution of HTTP requests in Java. It allows you to quickly create a request, add parameters, set content types, headers and more, execute it and handle the response and its data, both synchronously and asynchronously. While standardizing some aspects of the HTTP lifecycle for improved usability, the library still allows for a variety of options to be customized. Java-HTTP also supports the transmission of binary data over HTTP.
The package requires Java 8+
Contents
Guide
Download and import
You can automatically import Java-HTTP in your project using Maven or Gradle:
Maven:
<dependency> <groupId>com.raylabz</groupId> <artifactId>java-http</artifactId> <version>2.0.1</version> </dependency>
Gradle:
implementation 'com.raylabz:java-http:2.0.1'
Alternatively, you can download Java-HTTP as a .jar library:
Download .jarMaking an HTTP GET request
Java-HTTP allows you to make a simple GET request using:
BasicHTTPRequest.create("http://google.com", RequestMethod.GET) //Specify the URL and request method .build() //Build the request .sendAndWait(); //Send the request and wait for a response
Adding parameters
You can add parameters to your requests using the addParam() method, which supports various data types. This method expects the parameter name, and the value of the parameter.
BasicHTTPRequest.create("http://google.com", RequestMethod.GET) .addParam("intParam", 1) //Add an integer parameter .addParam("doubleParam", 3.14) //Add a double parameter .addParam("floatParam", 3.14f) //Add a float parameter .addParam("stringParam", "hello") //Add a String parameter .addParam("charParam", 'c') //Add a character parameter .addParam("booleanParam", true) //Add a boolean parameter .addParam("longParam", System.currentTimeMillis()) //Add a long parameter .addParam("shortParam", (short) 123) //Add a short parameter .build() .sendAndWait();
Handling the response
You can specify what happens when the response is received using the onSuccess() method and a lambda expression. The response object gives us access to important response information, such as the status, content and headers of the response.
BasicHTTPRequest.create("http://google.com", RequestMethod.GET) .onSuccess(response -> { System.out.println("Response received successfully"); //Print a message int status = response.getStatus(); //Get the status String body = response.getContent(); //Get the content List<String> keepAlive = response.getHeaderField("Keep-Alive"); //Get meta-data by name (headers) }) .build() .sendAndWait();
You can also specify what will happen when an error occurs during the execution of the request using the onFailure() method. This is different from the response status, as it indicates that an error has occurred on the client-side rather than on the server-side. Similar to onSuccess(), the onFailure() method can be defined using a lambda expression:
BasicHTTPRequest.create("http://google.com", RequestMethod.GET) .onFailure(error -> { error.printStackTrace(); }) .build() .sendAndWait();
Making an HTTP POST request
You can create and send a POST request or any other type of HTTP request (i.e. DELETE, PUT etc.) by changing the request method when creating a BasicHTTPRequest:
//POST request: BasicHTTPRequest.create("http://google.com", RequestMethod.POST) .build() .sendAndWait();
//PUT request: BasicHTTPRequest.create("http://google.com", RequestMethod.PUT) .build() .sendAndWait();
Defining request headers
You can define a header in your request using the setRequestProperty() method:
BasicHTTPRequest.create("http://google.com", RequestMethod.GET) .setRequestProperty("User-Agent", "Java") //Set the 'User-Agent' header .build() .sendAndWait();
Content type
You can define the content type for your request using the setContentType() method:
BasicHTTPRequest.create("http://google.com", RequestMethod.GET) .setContentType(ContentType.CONTENT_TYPE_JSON) .build() .sendAndWait();
Alternatively, if you want to provide a custom content type, you can use the setContentType() method and provide a string-based representation of the type:
BasicHTTPRequest.create("http://google.com", RequestMethod.GET) .setContentType("text/*") .build() .sendAndWait();
Timeouts
You can define the connection timeout and data read timeout limits (in milliseconds) using the setConnectTimeout() and setReadTimeout() methods:
BasicHTTPRequest.create("http://google.com", RequestMethod.GET) .setConnectTimeout(20000) //Set the connection timeout .setReadTimeout(15000) //Set the read data timeout .build() .sendAndWait();
Response status checks
You can quickly check if the status is successful by using the isSuccess() method:
BasicHTTPRequest.create("http://google.com", RequestMethod.GET) .onSuccess(response -> { if (response.isSuccess()) { //TODO - Success! } else { //TODO - Failed to connect :( } }) .build() .sendAndWait();
Asynchronous requests
An asynchronous request is a request which does not block the execution of the program, allowing other code to be executed concurrently with the request. Asynchronous requests can be made by using the send() method instead of sendAndWait().
BasicHTTPRequest.create("http://google.com", RequestMethod.GET) .build() .send(); //Send the request and continue executing other code
Binary requests
Java-HTTP allows you to easily create and send HTTP requests involving binary data. Binary requests and responses are ideal for sending larger chunks of data such as multimedia or documents, and communicating more efficiently. Binary requests send binary data and will always return binary responses, in raw bytes. They can be created using the BinaryHTTPRequest class:
BinaryHTTPRequest.create("http://localhost:8080/binaryEndpoint") .build() .sendAndWait();
You can put data into the request body using the following methods, which accept primitive types:
BinaryHTTPRequest.create("http://localhost:8080/binaryEndpoint") .putInt(1) //Put an integer .putShort((short) 1) //Put a short .putLong(System.currentTimeMillis()) //Put a long .putFloat(1.3f) //Put a float .putDouble(3.14) //Put a double .putChar('c') //Put a character .putString("hello") //Put a string .putBoolean(true) //Put a boolean .putByte((byte) 112) //Put a byte .putBytes(new byte[10]) //Put an array of bytes .build() .sendAndWait();
You can also use the same properties and methods as in simple HTTP requests:
BinaryHTTPRequest.create("http://localhost:8080/binaryEndpoint") .onSuccess(response -> { if (response.isSuccess()) { //... } }) .onFailure(error -> { error.printStackTrace(); }) .setConnectTimeout(10000) .setReadTimeout(15000) .setRequestProperty("User-Agent", "Java") .build() .send();
Handling binary responses
Binary responses can be handled using the onSuccess() method. Their content is in raw bytes, but the following methods can allow you to read primitive data types:
BinaryHTTPRequest.create("http://localhost:8080/binaryEndpoint") .onSuccess(response -> { if (response.isSuccess()) { int i = response.readInt(); long l = response.readLong(); short s = response.readShort(); float f = response.readFloat(); double d = response.readDouble(); char c = response.readChar(); String str = response.readString(); boolean b = response.readBoolean(); byte b1 = response.readByte(); } }) .build() .sendAndWait();
It is also possible to get the entire body of the response using response.getContent().
BinaryHTTPRequest.create("http://localhost:8080/binaryEndpoint") .onSuccess(response -> { if (response.isSuccess()) { final byte[] data = response.getContent(); } }) .build() .sendAndWait();
Latency
It is also possible to get the latency of a request-response cycle using the response.getLatency() method, which returns the latency in milliseconds:
BasicHTTPRequest.create("http://google.com", RequestMethod.GET) .onSuccess(response -> { long latency = response.getLatency(); }) .build() .sendAndWait();
Documentation
Visit the documentation.
License
Java-HTTP is released under the Apache 2.0 license.
Source code
You can find the source code at the project's repository here.
Bug reporting
Please report bugs here.