AsyncTask
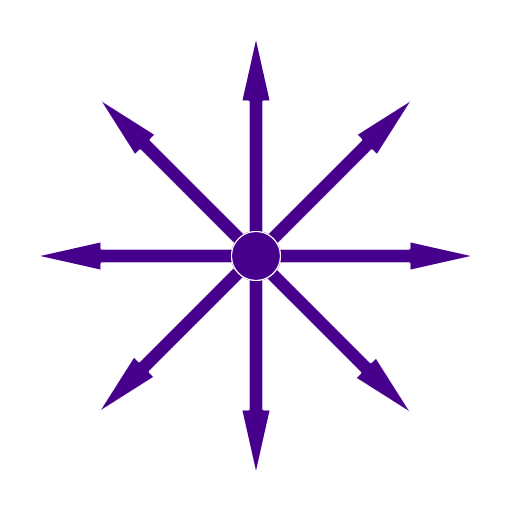
A port of Android SDK's AsyncTask for Java FX applications.
AsyncTask is a utility library that ports the Android SDK's AsyncTask class for use in Java FX applications.
It enables the easier use of threads in Java FX applications by allowing you to perform operations on non-UI threads and publish their results on the UI thread without having to deal with Threads, Executors and Handlers. AsyncTask does not follow any framework or specific SDK, so you can use it in any Java FX application.
The package requires Java 8+
Contents
Guide
Download and import
You can automatically import AsyncTask in your project using Maven or Gradle:
Maven:
<dependency> <groupId>com.raylabz</groupId> <artifactId>AsyncTask</artifactId> <version>1.1.0</version> </dependency>
Gradle:
implementation 'com.raylabz:AsyncTask:1.1.0'
Alternatively, you can download AsyncTask as a .jar library:
Download .jarCreating an AsyncTask
AsyncTask for Java is a simplified port of the Android SDK's version of AsyncTask. While it is designed to be very similar in use, it omits some functionality which is not frequently used. From this point, we are assuming that you already known the basics of AsyncTask usage from the Android SDK. Otherwise, you can refer to this guide by Alvin Alexander to learn how it works. You can use AsyncTask by creating a new class that extends the AsyncTask class:
public class MyAsyncTask extends AsyncTask<Void, Void, Void> { ... }
Note that the AsyncTask class takes three generic type arguments just like the Android AsyncTask, which are:
- Parameter type
- Progress type
- Result type
Implementing the methods
You can freely define your own methods and attributes in any AsyncTask you create. However, every class you create must implement the following three methods provided by the base class:
- onPreExecute()
- doInBackground()
- onPostExecute()
onPreExecute()
The onPreExecute() method executes code on the UI thread. It is usually used to prepare workload for execution while still being able to access the UI thread. An example would be URL sanitization, and initialization of certain UI elements. The following code shows an example implementation of the onPreExecute() method that prints a message on the console:
@Override protected void onPreExecute() { System.out.println("OnPreExecute" + System.lineSeparator()); }
doInBackground()
The doInBackground() method executes code on a non-UI thread - this is done to prevent the UI from freezing during long operations such as database queries, HTTP calls or file reads. The code below shows an example where numbers between 0 and 1,000,000 are printed on the screen - a long operation that would stall the application. This method receives a set of parameters and returns a result.
@Override protected Void doInBackground(Void... voids) { for (long i = 0; i < 1000000; i++) { System.out.println(i + System.lineSeparator()); } return null; }
onPostExecute()
The onPostExecute() method executes code on the UI thread and receives the result of the doInBackground() method.
@Override protected void onPostExecute(Void aVoid) { System.out.println("OnPostExecute" + System.lineSeparator()); }
Executing a task
You can execute an AsyncTask by using the execute() method:
asyncTask.execute();
If your AsyncTask accepts any parameters, you can pass them in the following way:
asyncTask.execute("param1", "param2", "param3");
Alternatively, you can use an array and pass the array as an argument:
String [] paramsArray = { "param1", "param2", "param3" }; asyncTask.execute(paramsArray);
Progress updates
You can update the progress indication on the UI while the task is running by using the methods publishProgress() and onProgressUpdate(). The publishProgress() method is used within doInBackground() to publish a set of Progress objects. Calling this method automatically invokes onProgressUpdate() on the UI thread, which you need to implement. onProgressUpdate() can be used to update the UI by using the progress provided by publishProgress(). The code below shows how to implement the onProgressUpdate() method and how to call the publishProgress() in doInBackground():
@Override protected void onProgressUpdate(Integer... integers) { super.onProgressUpdate(integers); System.out.println(integers[0] + System.lineSeparator()); } ... @Override protected Void doInBackground(Void... voids) { for (long i = 0; i < 1000000; i++) { stringBuilder.append("[" + name + "] -> " + i + System.lineSeparator()); if (i % 100000 == 0) { publishProgress((int) i); } } return null; }
Important note: Java FX applications will hang (not close) when using AsyncTask because AsyncTask utilizes the FX components to execute code on the main thread. To close your application, you will need to exit when a JFrame is closed using setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE) or explicitly exit your application at any time using PlatformImpl.tkExit() or System.exit().
Full example
Please see the complete example here.
Documentation
Visit the documentation.
License
AsyncTask for plain Java is released under the Apache 2.0 license.
Source code
You can find the source code at the project's repository here.
Bug reporting
Please report bugs here.