JSec
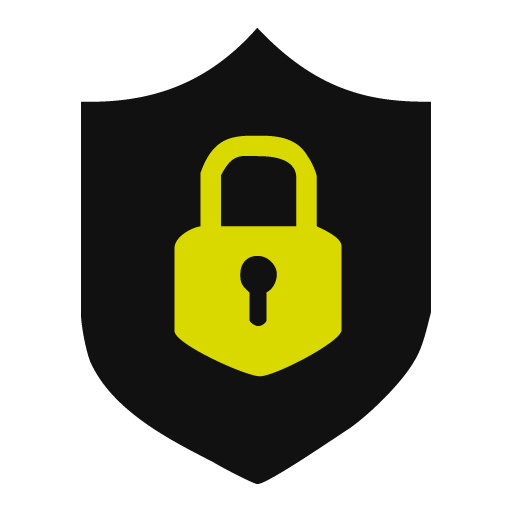
A simple encryption and hashing library for Java.
JSec is a simple security library which provides industry-standard encryption and hashing functions for Java applications. The library allows hashing using multiple algorithms and symmetric or asymmetric encryption. It is designed to be easy to use, speed up development time and allow developers to focus on other aspects of development rather than creating their own security packages.
The package requires Java 8+
Contents
Guide
Download and import
You can automatically import JSec in your project using Maven or Gradle:
Maven:
<dependency> <groupId>com.raylabz</groupId> <artifactId>jsec</artifactId> <version>1.0.0</version> </dependency>
Gradle:
implementation 'com.raylabz:jsec:1.0.0'
Alternatively, you can download JSec as a .jar library:
Download .jarHashing
JSec allows you to obtain a hash representation of text and raw data using various hashing algorithms.
SHA512 is used by default. To hash text or raw data, use the Hashing.hash() method.
final String input = "MyRandomInput"; String hash = Hashing.hash(input);
Alternatively, you can convert any type of data into raw bytes and hash them:
final String input = "MyRandomInput"; final byte[] rawData = input.getBytes(); String hash = Hashing.hash(rawData);
Hashing algorithms
You can also use other available hashing algorithms listed below (HashType), using the same function but providing the algorithm first:
- MD5
- SHA1
- SHA256
- SHA384
- SHA512 (default)
String hash = Hashing.hash(HashType.MD5, input);
However, use of the default hashing algorithm is suggested to provide better security. For even greater security, please look into using salt in the section below.
Hashing with salt
To make your hashes more secure, you need to use salt. Salt provides randomness to the algorithms, making the resulting hash computationally infeasible to guess or find using a rainbow table. A salt is a random string of bytes that randomizes the output of the algorithm. To provide better security, you need to create a salt and store it securely so that you can use it to re-create the same hash for the same data again if needed. To create a random salt, use the Hashing.salt() method:
final byte[] salt = Hashing.salt();
Then, you can pass the salt (pun intended) as a parameter to the hash() method:
Hashing.hash(input, salt); //OR Hashing.hash(HashType.MD5, input, salt);
Remember to store the salt in a file or database, otherwise you will not be able to re-create the same hash.
Symmetric encryption
You can symmetrically encrypt text with a key/password using the SymmetricEncryption class. This class utilizes the Advanced Encryption Standard (AES) algorithm, which is the adopted worldwide standard for symmetric encryption. To encrypt, use the SymmetricEncryption.encrypt() method with your input and key/password as parameters:
final String input = "MyRandomInput"; final String encrypted = SymmetricEncryption.encrypt(input, key);
To decrypt an encrypted text, you can use the decrypt() method:
final String decrypted = SymmetricEncryption.decrypt(encrypted, key);
Asymmetric Encryption
You can asymmetrically encrypt data using the AsymmetricEncryption class, which uses the RSA algorithm. Asymmetric encryption can be used to provide better security against password theft and is commonly used in secure communications. A public and private key are automatically generated for you, so you may directly encrypt data without having to create keys.
To asymmetrically encrypt data using the randomly generated keys, use the encrypt() method:
final String input = "MyRandomInput"; byte[] encrypted = AsymmetricEncryption.encrypt(input.getBytes());
You may then decrypt the data using the decrypt() method:
byte[] decrypted = AsymmetricEncryption.decrypt(encrypted);
Asymmetric encryption is useless without obtaining the public and private keys. To obtain these keys, use the getPrivateKey() and getPublicKey() methods correspondingly:
PrivateKey privateKey = AsymmetricEncryption.getPrivateKey(); PublicKey publicKey = AsymmetricEncryption.getPublicKey();
You may then convert these keys into bytes and store them in a file or database for future use or send them to another client, using the getEncoded() method:
byte[] privateKeyBytes = privateKey.getEncoded(); byte[] publicKeyBytes = publicKey.getEncoded();
After storing the keys, you can load them using the getPrivateKeyFromBytes() and getPublicKeyFromBytes() methods correspondingly:
privateKey = AsymmetricEncryption.getPrivateKeyFromBytes(privateKeyBytes); publicKey = AsymmetricEncryption.getPublicKeyFromBytes(publicKeyBytes);
Then use the setKeyPair() method to set the keys:
AsymmetricEncryption.setKeyPair(publicKey, privateKey);
In case you are loading pre-stored keys or received keys, make sure to load them before encrypting or decrypting, otherwise random keys will be used and the data will not be encrypted/decrypted correctly.
Full example
Please see the complete example here.
Documentation
Visit the documentation.
License
JSec is released under the Apache 2.0 license.
Source code
You can find the source code at the project's repository here.
Bug reporting
Please report bugs here.