EasyJSON
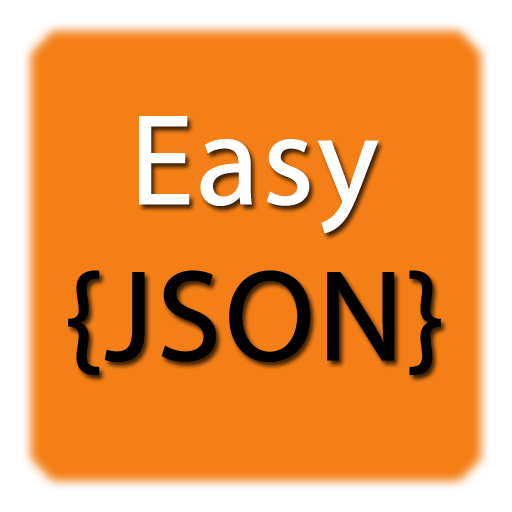
A simple JSON library for PHP
EasyJSON is a simple JSON library for PHP. It allows easy conversion and serialization of PHP objects and arrays to JSON format.
Supported PHP versions: 7.0+
Contents
Guide
Download and import
To begin, download EasyJSON:
Download
Place the EasyJSON folder in the root folder of your code and use the following code to import it in your PHP scripts:
require_once("EasyJSON\EasyJSON.php");
For simplicity, you can use PHP aliasing for JsonObject:
use EasyJSON\JsonObject as JsonObject;
Creating a custom class
A custom class can be converted to JSON format if it extends the JsonObject class. Therefore, all of the classes that are going to be converted to JSON format need to extend this class. The following example shows how to create an Address class. The class has four properties, road, number, state and country, along with a constructor and methods for setting and getting these properties.
use EasyJSON\JsonObject as JsonObject; class Address extends JsonObject { private $road; private $number; private $state; private $country; public function __construct($road, $number, $state, $country) { $this->road = $road; $this->number = $number; $this->state = $state; $this->country = $country; } public function getRoad() { return $this->road; } public function setRoad($road) { $this->road = $road; } public function getNumber() { return $this->number; } public function setNumber($number) { $this->number = $number; } public function getState() { return $this->state; } public function setState($state) { $this->state = $state; } public function getCountry() { return $this->country; } public function setCountry($country) { $this->country = $country; } }
If your custom class is declared in a separate file import it using:
require_once("Address.php");
You can instantiate an object of a custom class using:
$address = new Address("myRoad", 10, "myState", "myCountry");
Serialize/Encode to JSON text
You can use the toJSON() method to serialize/encode an object into its JSON text representation:
echo $address->toJson();
This will print: {"road":"myRoad","number":10,"state":"myState","country":"myCountry"}
Nesting objects
In some cases, you will need to create a custom object inside another object. To show an example, we will use a Person class, which will include the properties firstName, lastName, address and friends where the property address will be an object of class Address:
use EasyJSON\JsonObject as JsonObject; class Person extends JsonObject { private $firstName; private $lastName; private $address; //This property should contain an object of type Address. private $friends; public function __construct($firstName, $lastName, $address) { $this->firstName = $firstName; $this->lastName = $lastName; $this->address = $address; $this->friends = array(); } public function getFirstName() { return $this->firstName; } public function setFirstName($firstName) { $this->firstName = $firstName; } public function getLastName() { return $this->lastName; } public function setLastName($lastName) { $this->lastName = $lastName; } public function getAddress() { return $this->address; } public function setAddress($address) { $this->address = $address; } public function getFriends() { return $this->friends; } public function addFriend($friend) { array_push($this->friends, $friend); } }
Now, let us create a Person object that contains the address we have created before:
require_once("Person.php"); $person = new Person("John", "Doe", $address); echo $person->toJson();
This should print: {"firstName":"John","lastName":"Doe","address":{"road":"myRoad","number":10,"state":"myState","country":"myCountry"}}
Working with arrays
You can include arrays in your custom classes like in the Person class:
... private $firstName; private $lastName; private $address; //This property should contain an object of type Address. private $friends; public function __construct($firstName, $lastName, $address) { $this->firstName = $firstName; $this->lastName = $lastName; $this->address = $address; $this->friends = array(); } ... public function getFriends() { return $this->friends; } public function addFriend($friend) { array_push($this->friends, $friend); } ...
Let us create a second person, and assign it as a friend of the first person we have created:
$person2 = new Person("Jane", "Jones", $address); $person->addFriend($person2); echo $person->toJson();
This should print: {"firstName":"John","lastName":"Doe","address":{"road":"myRoad", "number":10,"state":"myState","country":"myCountry"},"friends":[{"firstName":"Jane","lastName":"Jones", "address":{"road":"myRoad","number":10,"state":"myState","country":"myCountry"}}]}
Let's add another person as friend to the first person:
$person3 = new Person("George", "Michaels", $address); $person->addFriend($person3); echo $person->toJson();
This should print: {"firstName":"John","lastName":"Doe","address":{"road":"myRoad", "number":10,"state":"myState","country":"myCountry"},"friends":[{"firstName":"Jane","lastName":"Jones", "address":{"road":"myRoad","number":10,"state":"myState","country":"myCountry"}},{"firstName":"George", "lastName":"Michaels","address":{"road":"myRoad","number":10,"state":"myState","country":"myCountry"}}]}
Full example
Please see the complete example here.
License
EasyJSON is released under the Apache 2.0 license.
Source code
You can find the source code at the project's repository here.
Bug reporting
Please report bugs here.